Snippet
indexByKey()
edit
0 strokes bestowed
const indexByKey = key => arr =>
arr.reduce((acc, cur) => {
acc[cur[key]] = cur
return acc
}, {})
Use this function to make look ups based on a key for an array of items O(1)
. So long as your list doesn’t change, you should only have to run this once.
Here’s an example:
const indexById = indexByKey('id')
const members = [
{ id: 1, name: 'Kyle' },
{ id: 2, name: 'Krios' }, // my cat
{ id: 3, name: 'Tali' }, // my other cat
]
const membersById = indexById(members)
/*
{
'1': { id: 1, name: 'Kyle' },
'2': { id: 2, name: 'Krios' },
'3': { id: 3, name: 'Tali' }
}
*/
Make sure the key you’re using is unique to each object, otherwise you’ll have an incorrect index due to key
collisions.
Liked the post?
Give the author a dopamine boost with a few "beard strokes". Click the beard
up to 50 times to show your appreciation.
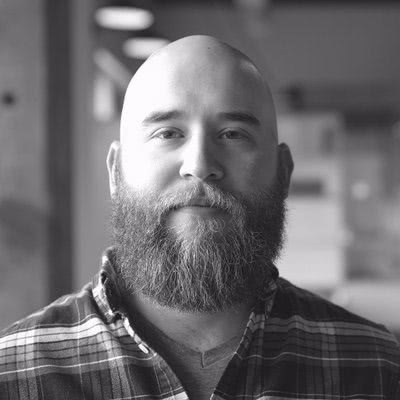
Kyle Shevlin is the founder & lead software engineer of Agathist, a software development firm with a mission to build good software with good people.